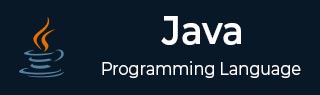
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
- Java - Number
- Java - Boolean
- Java - Characters
- Java - Strings
- Java - Arrays
- Java - Date & Time
- Java - Math Class
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Data Types
Data types define the type and value range of the data for the different types of variables, constants, method parameters, returns type, etc. The data type tells the compiler about the type of data to be stored and the required memory. To store and manipulate different types of data, all variables must have specified data types.
Java data types are categorized into two parts −
- Primitive Data Types
- Reference/Object Data Types
Java Primitive Data Types
Primitive data types are predefined by the language and named by a keyword. There are eight primitive data types supported by Java. Below is the list of the primitive data types:
- byte
- short
- int
- long
- float
- double
- boolean
Java byte Data Type
Byte data type is an 8-bit signed two's complement integer
Minimum value is -128 (-2^7)
Maximum value is 127 (inclusive)(2^7 -1)
Default value is 0
Byte data type is used to save space in large arrays, mainly in place of integers, since a byte is four times smaller than an integer.
Example − byte a = 100, byte b = -50
Java short Data Type
Short data type is a 16-bit signed two's complement integer
Minimum value is -32,768 (-2^15)
Maximum value is 32,767 (inclusive) (2^15 -1)
Short data type can also be used to save memory as byte data type. A short is 2 times smaller than an integer
Default value is 0.
Example − short s = 10000, short r = -20000
Java int Data Type
Int data type is a 32-bit signed two's complement integer.
Minimum value is - 2,147,483,648 (-2^31)
Maximum value is 2,147,483,647(inclusive) (2^31 -1)
Integer is generally used as the default data type for integral values unless there is a concern about memory.
The default value is 0
Example − int a = 100000, int b = -200000
Java long Data Type
- Long data type is a 64-bit signed two's complement integer
- Minimum value is -9,223,372,036,854,775,808(-2^63)
- Maximum value is 9,223,372,036,854,775,807 (inclusive)(2^63 -1)
- This type is used when a wider range than int is needed
- Default value is 0L
- Example − long a = 100000L, long b = -200000L
Java float Data Type
Float data type is a single-precision 32-bit IEEE 754 floating point
Float is mainly used to save memory in large arrays of floating point numbers
Default value is 0.0f
Float data type is never used for precise values such as currency
Example − float f1 = 234.5f
Java double Data Type
double data type is a double-precision 64-bit IEEE 754 floating point
This data type is generally used as the default data type for decimal values, generally the default choice
Double data type should never be used for precise values such as currency
Default value is 0.0d
Example − double d1 = 123.4
Java boolean Data Type
boolean data type represents one bit of information
There are only two possible values: true and false
This data type is used for simple flags that track true/false conditions
Default value is false
Example − boolean one = true
Java char Data Type
char data type is a single 16-bit Unicode character
Minimum value is '\u0000' (or 0)
Maximum value is '\uffff' (or 65,535 inclusive)
Char data type is used to store any character
Example − char letterA = 'A'
Example: Demonstrating Different Primitive Data Types
Following examples shows the usage of variour primitive data types we've discussed above. We've used add operations on numeric data types whereas boolean and char variables are printed as such.
public class JavaTester { public static void main(String args[]) { byte byteValue1 = 2; byte byteValue2 = 4; byte byteResult = (byte)(byteValue1 + byteValue2); System.out.println("Byte: " + byteResult); short shortValue1 = 2; short shortValue2 = 4; short shortResult = (short)(shortValue1 + shortValue2); System.out.println("Short: " + shortResult); int intValue1 = 2; int intValue2 = 4; int intResult = intValue1 + intValue2; System.out.println("Int: " + intResult); long longValue1 = 2L; long longValue2 = 4L; long longResult = longValue1 + longValue2; System.out.println("Long: " + longResult); float floatValue1 = 2.0f; float floatValue2 = 4.0f; float floatResult = floatValue1 + floatValue2; System.out.println("Float: " + floatResult); double doubleValue1 = 2.0; double doubleValue2 = 4.0; double doubleResult = doubleValue1 + doubleValue2; System.out.println("Double: " + doubleResult); boolean booleanValue = true; System.out.println("Boolean: " + booleanValue); char charValue = 'A'; System.out.println("Char: " + charValue); } }
Output
Byte: 6 Short: 6 Int: 6 Long: 6 Float: 6.0 Double: 6.0 Boolean: true Char: A
Java Reference/Object Data Type
The reference data types are created using defined constructors of the classes. They are used to access objects. These variables are declared to be of a specific type that cannot be changed. For example, Employee, Puppy, etc.
Class objects and various types of array variables come under reference datatype. The default value of any reference variable is null. A reference variable can be used to refer to any object of the declared type or any compatible type.
Example
The following example demonstrates the reference (or, object) data types.
// Creating an object of 'Animal' class Animal animal = new Animal("giraffe"); // Creating an object of 'String' class String myString = new String("Hello, World!");
To Continue Learning Please Login