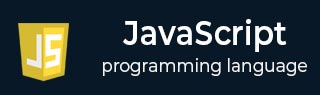
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Modules
What is a Module?
A module in JavaScript is a single file containing a JavaScript code or script. Rather than adding the code for the whole application in a single file, we can break down the code and create separate files for the code having the same functionalities.
In short, the module contains the code to perform the specific task. It can contain variables, functions, classes, etc.
When the size of the application grows, number of lines in the code also grows. Even real-time applications contain millions of lines of code. Now, think about if developers have only a single file containing millions of lines of code. Are they able to manage it properly? The simple answer is no. Here, using the module comes into the picture.
Syntax
Users can follow the syntax below to export and import the modules.
export function func_name(parameters) { // function code } import {func_name} from filename
In the above syntax, the export keyword is used to export the function from the module, and the import keyword is used to import the function from the module.
Example
Filename: mul.js
In the code below, we defined the 'mul()’ function in the 'mul.js' file, which takes two integers as a parameter and returns the multiplication of them. Also, we used the 'export' keyword before the 'function' keyword to export the function from the module.
// exporting mul() function export function mul(a, b) { return a * b; }
Filename: test.html
In the above code, we have imported the module in an HTML file. However, we can also import the module in another JavaScript file. You can also export multiple variables, functions, etc., from a single module. Let's understand it via the example below. FileName: math.js In the code below, we have exported the mul() and sum() functions. Also, we have exported the variable containing the value of PI. In short, we are exporting the multiple objects from the single module. FileName: test.html In the code below, we import multiple objects from a single module and use them to perform the mathematical operations. You can use the 'default' keyword to define the default export in the module. After that, whenever you try to import the object that is not defined in the module, it returns the object that is exported by default. The default export helps in avoiding the import error. Users can follow the syntax below to use the default exports in the module. In the above syntax, the 'default' keyword is used after the export keyword to export the 'func_name' function by default. FileName: test.js In the code below, we export the 'v1' variable by default, and we also export the 'v2' variable. FileName: test.html In the code below, we have imported the 'v3' and 'v2' from the test.js file. As 'v3' is not exported from the test.js file, it imports the default object, which is 'v1’. Sometimes, it happens that the code file and module file contain the same name of the object. In such cases, if the developer imports the module in the code file, it can raise conflicts and errors. To resolve this issue, we can change the name of the module either while exporting or importing. Users can follow the syntax below to rename the exports. In the above syntax, we have changed the name of func1 to N1 and func2 to N2 at the time of exporting from the module. We used the 'as' keyword to change the name of the function. Users can follow the syntax below to rename the imports. In the above syntax, we change the function name while importing it.
<html>
<body>
<div> JavaScript - Modules </div>
<div id = "output"> </div>
<script type="module">
import { mul } from './mul.js';
document.getElementById('output').innerHTML =
"The multiplication of 2 and 3 is " + mul(2, 3);
</script>
</body>
</html>
Output
JavaScript - Modules
The multiplication of 2 and 3 is 6
Export Multiple Objects from a Single Module
Example
// exporting mul() function
export function mul(a, b) {
return a * b;
}
// exporting sum() function
export function sum(a, b) {
return a + b;
}
// export value of PI
export let PI = Math.PI;
<html>
<body>
<div> Exporting Multiple Objects from a Single Module </div>
<div id = "output"> </div>
<script type="module">
import { mul, sum, PI } from './math.js';
document.getElementById('output').innerHTML =
"The multiplication of 2 and 3 is " + mul(2, 3 +
"<br> The addition of 2 and 3 is " + sum(2, 3) +
"<br> The value of PI is " + PI;
</script>
</body>
</html>
Output
Exporting Multiple Objects from a Single Module
The multiplication of 2 and 3 is 6
The addition of 2 and 3 is 5
The value of PI is 3.141592653589793
Default Exports
Syntax
export default function func_name(params) {
// function body
}
import random_name from filename;
Example
const v1 = "Version 1";
// default export
export default v1;
// other modules
export const v2 = "Version 2";
<html>
<body>
<div> Default Export </div>
<div id = "output"> </div>
<script type="module">
// V3 is not defined in the module, so it returns V1.
import v3, { v2 } from './test.js';
document.getElementById('output').innerHTML =
"The value of v3 is : " + v3 + "<br>" +
"The value of v2 is : " + v2;
</script>
</body>
</html>
Output
Default Export
The value of v3 is : Version 1
"The value of v2 is : Version 2
Rename Import and Export
Syntax to Rename Exports
export {
func1 as N1,
func2 as N2
};
// import in the code file
import { N1, N2 } from './module.js';
Syntax to Rename Imports
export {
func1,
func2
};
// import in the code file
import { func1 as N1, func1 as N2 } from './module.js';
To Continue Learning Please Login