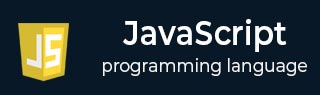
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Unicode
What is Unicode?
Unicode is a universal set of characters that contains a list of characters from the majority of languages, writing systems, etc. It provides a unique number for every character without focusing on programming language, platform, operating system, etc. Furthermore, it also includes punctuation, emojis, special characters, etc.
In short, the Unicode set contains unique numbers, each referring to a unique character, having the same meaning regardless of platform, operating system, etc.
Intuition behind Unicode
Before understanding unicode, let's understand the idea behind it. Can you answer the question of why are you able to read this tutorial? Well, because you know the meaning of the letters written. A reader (you) and writer both have the same comprehension of the English alphabetical letters; that's why you are able to read what the writer has written.
Similarly, computers don't understand the letters. For computers, letters are sequences of bits, and each sequence is mapped to a unique character that is called Unicode.
Now, let's understand Unicode in depth.
Unicode in JavaScript
JavaScript allows developers to use the Unicode characters in the string literals and source code. Developers need to use the escape notation (\u) to use the Unicode characters in JavaScript code.
Syntax
Users can follow the syntax below to use the Unicode characters in JavaScript.
const char = '\uxxxx';
In the above syntax, '\uxxxx' is a Unicode character. Here, 'xxxx' represents the hexadecimal characters, and β/u' represents the escape notation.
Examples
Example: Unicode escape sequence
In the below example, we have used unicode escape sequence to print the "hello" message.
<html> <body> <div>Using unicode escape sequence</div> <div id = "output"> </div> <script> let str = '\u0068\u0065\u006c\u006c\u006f' document.getElementById("output").innerHTML = str; </script> </body> </html>
Output
Using unicode escape sequence hello
Example: Using unicode characters in variable names
In the code below, we have used the two different Unicode characters as two different identifiers (variable names). In the output, you can observe the value of both identifiers.
<html> <body> <div>Using unicode characters in variable names</div> <div id = "output"> </div> <script> // Using the Unicode characters in variable names let \u0061 = "Hello"; let \u0062 = "World"; document.getElementById("output").innerHTML = a + " " + b; </script> </body> </html>
Output
Using unicode characters in variable names Hello World
Example: Using the Unicode Characters in String
In this example, we have used the Unicode characters in the string literals. The output shows the special characters in the middle of the string.
<html> <body> <div> Using the Unicode Characters in String </div> <div id = "output"> </div> <script> // Using the Unicode characters in the string let str = 'Hello \u00D8 \u00F8 World'; document.getElementById("output").innerHTML = str; </script> </body> </html>
Output
Using the Unicode Characters in String Hello Γ ΓΈ World
Example: Using Unicode for non-BMP (Basic Multilingual Plane) characters
In the below example we have used unicode characters (code points) to show a non-BMP (basic multilingual plane) characters. We have demonstrate for a health worker.
<html> <body> <div>showing person heath worker using unicode code point</div> <div id = "output"> </div> <script> // Showing emojis using the unicode characters const smileyFace = '\u{1F9D1}\u200D\u2695\uFE0F'; document.getElementById("output").innerHTML = smileyFace; </script> </body> </html>
Output
showing person heath worker using unicode code point π§ββοΈ
Example: Showing Emojies Using the Unicode Characters
In the code below, we have used the Unicode character to show the smiley face emoji.
<html> <body> <div>Showing Emojies Using the Unicode Characters </div> <div id = "output"> </div> <script> // Showing emojis using the unicode characters const smileyFace = '\uD83D\uDE0A'; document.getElementById("output").innerHTML = smileyFace; </script> </body> </html>
Output
Showing Emojies Using the Unicode Characters π
As we have seen, each Unicode character represents a unique character. In JavaScript, we can use Unicode characters with identifiers, string literals, etc.
To Continue Learning Please Login