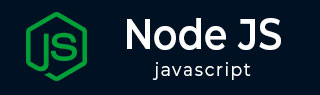
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Built-in Modules
A module in Node.js is a collection of independent and reusable code that can be imported into any Node.js application. The Node.js runtime software comes with the V8 JavaScript engine, bundled with a number of core modules, that perform important server-side tasks, such as managing event loop, perform file IO and operating system-specific functions etc.
Example
The following code snippet returns a list of all the built-in modules −
const builtinModules = require('repl')._builtinLibs; console.log(builtinModules);
Output
[ 'assert', 'assert/strict', 'async_hooks', 'buffer', 'child_process', 'cluster', 'console', 'constants', 'crypto', 'dgram', 'diagnostics_channel', 'dns', 'dns/promises', 'domain', 'events', 'fs', 'fs/promises', 'http', 'http2', 'https', 'inspector', 'inspector/promises', 'module', 'net', 'os', 'path', 'path/posix', 'path/win32', 'perf_hooks', 'process', 'punycode', 'querystring', 'readline', 'readline/promises', 'repl', 'stream', 'stream/consumers', 'stream/promises', 'stream/web', 'string_decoder', 'sys', 'timers', 'timers/promises', 'tls', 'trace_events', 'tty', 'url', 'util', 'util/types', 'v8', 'vm', 'wasi', 'worker_threads', 'zlib' ]
Node.js has many core modules that provide essential functionalities for building applications. Here's a list of some of the most important core modules −
Sr.No | Core Modules & Description |
---|---|
1 | http Provides an interface for creating HTTP servers and making HTTP requests. |
2 | fs Provides functions for working with files and directories. |
3 | path Provides functions for working with file paths. |
4 | url Provides functions for parsing and building URLs. |
5 | util Provides utility functions for working with data and strings. |
6 | crypto Provides functions for cryptography and secure hashing. |
7 | process Provides information about the current Node.js process and allows you to interact with the operating system. |
8 | net Provides low-level networking functionality. |
9 | stream Provides a basic framework for working with streams of data. |
10 | events Provides an event emitter class for custom event handling. |
11 | console Provides functions for writing to the console. |
12 | readline Provides functions for reading line-by-line from a stream. |
Select a module to see a list of all the methods related to that module −
To Continue Learning Please Login